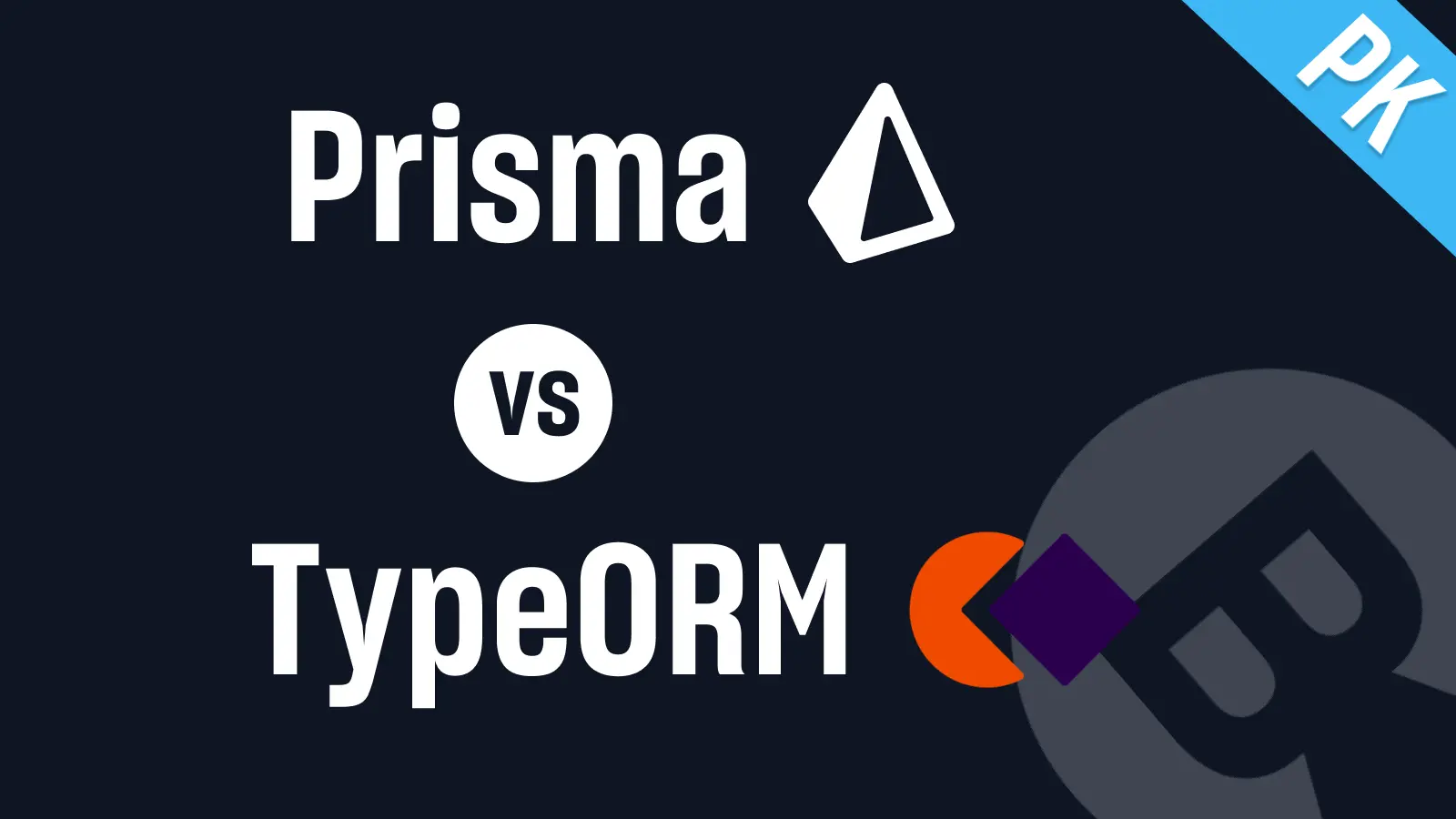
This post is maintained by Bytebase, an open-source database DevSecOps tool. We update the post every year.
Update History | Comment |
---|---|
2025/05/23 | Initial version. |
Prisma ORM
Prisma is a widely-used, full-featured ORM for Node.js and TypeScript with a strong focus on type safety, developer experience, and declarative schema design.
Core Components:
- Prisma Client: Auto-generated, type-safe query builder based on your data model.
- Prisma Migrate: Declarative schema migrations using .prisma files.
- Prisma Studio: GUI for viewing/editing data (for local use, not open-source).
Key Features:
- Schema-first workflow: Define models in Prisma Schema Language (PSL).
- Broad database support: PostgreSQL, MySQL, SQL Server, SQLite, MongoDB, and more.
- Type-safe and ergonomic: Tight IDE integration and error handling.
- Raw SQL support: When needed, bypass the abstraction.
- Tools for scaling: Prisma Accelerate (connection pooling, caching), Prisma Pulse (real-time events).
- Introspection: Generate schema from an existing database.
Prisma is great for teams that want an easy, type-safe ORM with strong tooling and rich ecosystem support.
TypeORM
TypeORM is a mature and versatile ORM built for TypeScript and JavaScript applications. It stands out for its flexibility, offering both Active Record and Data Mapper patterns, making it adaptable to various project architectures and developer preferences.
Key Features:
- Pattern Flexibility: Only JS/TS ORM supporting both Active Record and Data Mapper.
- Multi-Platform: Runs on Node.js, browsers, mobile (React Native, Ionic, Expo), and desktop (Electron).
- SQL & NoSQL: Supports MySQL, PostgreSQL, MongoDB, SQLite, MS SQL, and more.
- Data Modeling: Entities, relationships (eager/lazy), cascades, indices, inheritance.
- Database Tools: Migrations, transactions, replication, connection pooling, cross-database queries.
- Query Builder: Joins, pagination, caching, raw streaming, closure table pattern.
- Extras: CLI, logging, hooks (listeners/subscribers), TypeScript/ESM/CommonJS support.
TypeORM is ideal for developers who value flexibility, SQL control, and a mature ecosystem with comprehensive features.
Prisma ORM vs. TypeORM
Here's a comprehensive comparison of these two leading TypeScript ORMs:
Feature | Prisma ORM | TypeORM |
---|---|---|
Philosophy | Schema-first, declarative approach | SQL-friendly, flexible patterns |
Type Safety | Excellent via generated Prisma Client | Strong via TypeScript decorators |
Schema | In .prisma file (PSL) | In TypeScript using decorators |
Migrations | Declarative and integrated via Prisma Migrate | Manual or CLI-based |
Querying | Abstracted API with optional raw SQL | SQL-like query builder |
Performance | Good with some overhead from query engine | Good overall, SQL-optimized |
Serverless | Better with Prisma Accelerate | Requires connection management |
Tooling | Client, Studio, Accelerate, Pulse | CLI and various utilities |
Learning Curve | Lower for abstraction-oriented devs | Steeper for SQL beginners |
DB Support | mainstream databases but not legacy ones | include legacy databases |
Code Gen | Heavy (client generation from schema) | Minimal (decorators-based) |
Community | Growing rapidly | Large and established |
Open Source | Most tools OSS, Studio is local-only and closed | All components |
Philosophy & Design
Prisma ORM uses a schema-first approach with its own schema language. It hides SQL complexity behind a type-safe, auto-generated client. Prisma focuses on developer experience, making it easy for those who prefer working with objects over raw SQL.
TypeORM takes a SQL-friendly, flexible approach. It supports both Active Record and Data Mapper patterns, offering more control for developers familiar with SQL. It aims to stay close to the database while using TypeScript for type safety.
Type Safety
Prisma ORM offers strong, automatic type safety through its generated client. All queries—simple or complex—are fully type-checked based on your schema, which helps prevent runtime errors and boosts developer confidence.
TypeORM uses TypeScript decorators and interfaces for type safety. It covers most cases at compile time but may miss some complex queries. You need to be careful to keep everything properly typed.
Schema Definition & Migrations
Prisma uses a dedicated schema language in .prisma
files. This approach provides a clear, declarative way to define your data model separate from your application code. Prisma Migrate offers a more integrated migration experience, automatically generating and applying SQL migrations based on schema changes. This declarative approach simplifies the migration process, especially for teams managing complex schemas.
// Prisma schema definition
model User {
id Int @id @default(autoincrement())
email String @unique
name String?
posts Post[]
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
model Post {
id Int @id @default(autoincrement())
title String
content String?
published Boolean @default(false)
author User @relation(fields: [authorId], references: [id])
authorId Int
createdAt DateTime @default(now())
updatedAt DateTime @updatedAt
}
TypeORM defines schemas using TypeScript classes with decorators. This approach feels natural to TypeScript developers and keeps the schema definition within the codebase. For migrations, TypeORM offers both automatic generation and manual creation options, but the process can sometimes require manual intervention to handle complex changes.
// TypeORM schema definition
import { Entity, PrimaryGeneratedColumn, Column, OneToMany } from "typeorm"
import { Post } from "./Post"
@Entity()
export class User {
@PrimaryGeneratedColumn()
id: number
@Column()
email: string
@Column({ nullable: true })
name: string
@OneToMany(() => Post, post => post.author)
posts: Post[]
@Column({ type: 'timestamp', default: () => 'CURRENT_TIMESTAMP' })
createdAt: Date
@Column({
type: 'timestamp',
default: () => 'CURRENT_TIMESTAMP',
onUpdate: 'CURRENT_TIMESTAMP'
})
updatedAt: Date
}
Query Building & Raw SQL
Prisma offers an abstracted, object-oriented query API via the generated Prisma Client. This approach hides SQL complexity while still providing powerful querying capabilities. The API is designed to be intuitive and productive, with excellent autocompletion support. For cases where the abstraction is limiting, Prisma supports raw SQL queries via $queryRaw
and $executeRaw
.
// Prisma query examples
// Find users with filtering
const users = await prisma.user.findMany({
where: {
age: {
gt: 18
},
firstName: {
contains: 'john'
}
},
orderBy: {
lastName: 'asc'
},
take: 10,
skip: 5
});
// Query with relations
const postsWithComments = await prisma.post.findMany({
where: {
published: true,
comments: {
some: {
approved: true
}
}
},
include: {
comments: {
where: {
approved: true
}
}
}
});
// Raw SQL
const users = await prisma.$queryRaw`
SELECT * FROM users WHERE age > ${18} AND first_name LIKE ${"%john%"}
`;
TypeORM provides a SQL-like query builder that closely mirrors SQL syntax using method chaining. This approach is intuitive for developers familiar with SQL and offers fine-grained control over query construction. Raw SQL is easily integrated when needed, giving developers full access to database-specific features.
// TypeORM query examples
// Find users with filtering
const users = await userRepository.find({
where: {
age: MoreThan(18),
firstName: Like('%john%')
},
order: {
lastName: "ASC"
},
take: 10,
skip: 5
});
// Complex query with joins
const postsWithComments = await postRepository
.createQueryBuilder("post")
.leftJoinAndSelect("post.comments", "comment")
.where("post.isPublished = :isPublished", { isPublished: true })
.andWhere("comment.isApproved = :isApproved", { isApproved: true })
.getMany();
// Raw SQL
const users = await userRepository.query(
"SELECT * FROM users WHERE age > $1 AND first_name LIKE $2",
[18, "%john%"]
);
Performance & Bundle Size
Prisma uses a Rust-based query engine that adds some overhead but provides consistent performance. The bundle size is larger due to this engine, which can impact cold starts in serverless environments. To address this, Prisma offers Prisma Accelerate, a connection pooling and caching service specifically designed to improve performance in serverless contexts.
TypeORM is generally performant for most applications, with direct SQL translation that minimizes overhead. Its bundle size is moderate, making it suitable for various deployment environments. However, it doesn't have specific optimizations for serverless environments, which may require additional configuration for optimal performance.
Ecosystem & Tooling
Prisma offers a rich ecosystem of tools including Prisma Client, Prisma Migrate, Prisma Studio for visual database management, Prisma Accelerate for connection pooling and caching, and Prisma Pulse for real-time events. This comprehensive tooling creates a cohesive development experience, particularly valuable for teams that want an integrated solution.
TypeORM provides a CLI for various tasks and integrates well with the broader TypeScript ecosystem. Its tooling is functional but more basic compared to Prisma. The ecosystem includes various community-created extensions and plugins.
Learning Curve
Prisma offers a gentler learning curve, particularly for developers new to database interactions. Its abstracted API and clear documentation make it accessible, while the schema-first approach provides a clear mental model. The strong tooling and IDE integration further flatten the learning curve by providing helpful guidance during development.
TypeORM has a steeper learning curve, especially for developers less familiar with SQL or ORM concepts. Its flexibility means there are multiple ways to accomplish tasks, which can be overwhelming for beginners. However, developers with SQL experience will find its approach familiar and intuitive.
Database Support
Prisma supports PostgreSQL, MySQL, MariaDB, SQL Server, SQLite, CockroachDB, and MongoDB (with some limitations). While its database support is broad, it may not cover some specialized or legacy database systems that TypeORM supports.
TypeORM supports a wide range of databases including MySQL, MariaDB, PostgreSQL, CockroachDB, SQLite, Microsoft SQL Server, Oracle, SAP Hana, and MongoDB. This extensive support makes it versatile for various project requirements and legacy systems.
Code Generation
Prisma relies heavily on code generation, creating a Prisma Client based on your schema. This approach produces more generated code but enables the exceptional type safety that Prisma is known for. The generated client needs to be updated whenever the schema changes, adding an extra step to the development workflow.
TypeORM uses a minimal code generation approach based on decorators and TypeScript metadata. This approach keeps the codebase cleaner with less generated code to manage, but it may provide less comprehensive type safety compared to Prisma.
Community & Open Source
Prisma has a rapidly growing community with excellent official documentation and learning resources. Most components are open-source, but Prisma Studio is local-only and not open-source, which may be a consideration for teams that prioritize fully open solutions.
TypeORM has a large, established community with extensive documentation and third-party resources. All components are fully open-source, allowing for complete transparency and community contributions.
Conclusion
Choose Prisma if you prioritize developer productivity, strong type safety, and a clean, declarative development experience. Its intuitive API and robust tooling make it a great fit for modern applications where rapid development and reliability are key.
Opt for TypeORM if you need fine-grained control over SQL, support for a wider range of databases, or prefer working closer to the metal with a flexible ORM. It’s ideal for teams comfortable with SQL who want maximum customization and architectural freedom.